How to use Iterator in Java - Iterator Examples with List, Set, Map and Queue
- Details
- Written by Nam Ha Minh
- Last Updated on 11 April 2024   |   Print Email
You know, there are several ways to iterate collections in Java, but using iterator is the most basic and original. And any Java programmers should be familiar with and fluent in using iterator.
Table of content:1. Why using Iterator?
2. The Iterator Interface
3. Iterate a List
4. Iterate a Set
5. Iterate a Map
6. Iterate a Queue
7. Iterate a General Collection
1. Why using Iterator?
Iterator can be used for trivial traversals over elements in any collections with standard methods hasNext() and next(), plus it’s more convenient when you need to remove elements during iteration, using remove() method. So consider using iterator if:- You don’t know what the actual type of the collection will be. In other words, the type of the collection is not known at compile time. It can be List, Set or Queue at runtime.- You need to modify the collection (remove specific elements) while iterating.
2. The Iterator Interface
The following class diagram illustrates how the Iterator interface relates to others in Java Collections framework, as well as Iterator’s methods (APIs):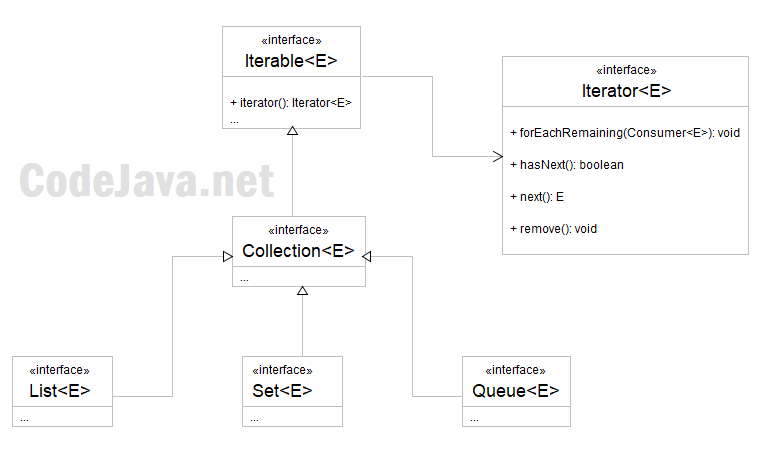
- hasNext(): returns true if the iteration has more elements
- next(): returns the next element in the iteration. It throws NoSuchElementException if the iteration has no more elements.
- remove(): removes the last element returned by this iterator from the underlying collection. This operation is optional and not supported by all collections (it will throw UnsupportedOperationException if not implemented).
- forEachRemaining(Consumer<? Super E> action): performs the given action for each remaining element until all elements have been processed or the action throws an exception. This method was added from Java 1.8.
3. Iterate a List
Given the following List collection created with some fixed elements:List<String> listFruits = List.of("Apple", "Banana", "Lemon", "Dragon Fruit", "Durian");The following code will iterate over all elements and print each, using a while loop:
Iterator<String> iterator = listFruits.iterator(); while (iterator.hasNext()) { String aFruit = iterator.next(); System.out.println(aFruit); }It’s also possible to use a for loop with an iterator, as shown below:
for ( ; iterator.hasNext(); ) { String aFruit = iterator.next(); System.out.println(aFruit); }If the list is mutable, we can remove elements from it. For example, the following code will remove String elements starting with “D”:
while (iterator.hasNext()) { String aFruit = iterator.next(); if (aFruit.startsWith("D")) iterator.remove(); }If the list is immutable, you will get UnsupportedOperationException.
4. Iterate a Set
Similar to List, elements in a Set can be iterated using iterator, as illustrated in the following code example:Set<Integer> numbers = new HashSet<>(Set.of(1, 2, 3, 4, 5, 6, 7, 8)); Iterator<Integer> iterator = numbers.iterator(); while (iterator.hasNext()) { Integer nextNumber = iterator.next(); System.out.println(nextNumber); }This example creates a set with some fixed elements, and use iterator to go through all elements, and print out value of each number in the set. Note that Sets do not order elements so there’s no guarantee about the order of elements returned by the iterator.
5. Iterate a Map
Maps store elements in terms of key-value pairs so it doesn’t implement Collection. However, we can iterate over a Map by getting iterator from its key set. The following code snippet gives you the idea:Map<Integer, String> map = Map.of(200, "OK", 404, "Not Found", 500, "Internal Server Error"); Iterator<Integer> keyIterator = map.keySet().iterator(); while (keyIterator.hasNext()) { Integer nextKey = keyIterator.next(); String nextValue = map.get(nextKey); System.out.println(nextKey + " => " + nextValue); }Here, the keyIterator object is of a Set collection that contains keys in the map. It is not iterator of the Map itself.
6. Iterate a Queue
Queues do implement Collection interface, so we can use iterator to traverse a queue as easy as shown in the following code example:Queue<String> queue = new PriorityQueue<>(Arrays.asList("One", "Two", "Three", "Four")); Iterator<String> iterator = queue.iterator(); while (iterator.hasNext()) { String next = iterator.next(); System.out.println(next); }
7. Iterate a General Collection
If the actual collection type is not known at compile time, it’s convenient to use iterator to traverse elements. Let’s see the following example:void process(Collection<String> collection) { Iterator<String> iterator = collection.iterator(); while (iterator.hasNext()) { String next = iterator.next(); // process the next element returned by the iterator System.out.println(next); } }You see, this method uses a parameter of type Collection, which means it can accept List, Set or Queue. NOTES: The Iterator interface defines the forEachRemaining() method which can be used to iterate all elements in a collection, as shown the following code:
List<String> listFruits = List.of("Apple", "Banana", "Lemon", "Dragon Fruit", "Durian"); listFruits.iterator().forEachRemaining(System.out::println);This method takes a parameter of type Consumer, which means you can pass a Lambda expression that results an object implementing that functional interface.I hope you find this article helpful, in terms of understanding how to use iterator in the Java Collections framework.
Reference:
Other Java Collections Tutorials:
- What is Java Collections Framework?
- Java Queue Tutorial
- Java List Tutorial
- Java Set Tutorial
- Java Map Tutorial
- Understand equals and hashCode
- Understand object ordering
- 18 Java Collections and Generics Best Practices
About the Author:

Comments