JLabel basic tutorial and examples
- Details
- Written by Nam Ha Minh
- Last Updated on 06 July 2019   |   Print Email
1. Creating a JLabel object
- Create a basic label with some text:
JLabel label = new JLabel("This is a basic label");
Image: - Create a label with empty text and set the text later:
JLabel label = new JLabel(); label.setText("This is a basic label");
- Create a label with only an icon (the icon file is in the file system and relative to the program):
JLabel label = new JLabel(new ImageIcon("images/attention.jpg"));
Image:This is the common way to display an image/icon in Swing.
- Create a label with only an icon (the icon file is in the classpath or in a jar file):
String iconPath = "/net/codejava/swing/jlabel/Color.png"; Icon icon = new ImageIcon(getClass().getResource(iconPath)); JLabel label = new JLabel(icon);
- Create a label with both text and icon and horizontal alignment is center:
JLabel label = new JLabel("A label with icon and text", new ImageIcon("images/attention.jpg"), SwingConstants.CENTER);
Image:
In this case, we can set the gap between the icon and the text as follows:label.setIconTextGap(10);
That will set 10 pixels gap between the icon and the text. - We can also specify horizontal alignment of the text/icon when creating the label:
JLabel label = new JLabel("Enter first name:", SwingConstants.RIGHT); JLabel label = new JLabel(new ImageIcon("images/attention.jpg"), SwingConstants.LEFT);
2. Adding the label to a container
- A JLabel is usually added to a JPanel, a JFrame, a JDialog or a JApplet:
frame.add(label); dialog.add(label); panel.add(label); applet.getContentPane().add(label);
- Adding a JLabel to a container with a specific layout manager:
frame.add(label, BorderLayout.CENTER); panel.add(label, gridbagConstraints);
3. Customizing JLabel’s appearance
- Change font style, background color and foreground color of the label:
label.setFont(new java.awt.Font("Arial", Font.ITALIC, 16)); label.setOpaque(true); label.setBackground(Color.WHITE); label.setForeground(Color.BLUE);
Image:NOTE:by default, the label’s background is transparent, so if you want to set background, you have to set the label’s opaque property to true.
- Instead of using the methods above, we can use HTML code to customize the label’s appearance. For example:
label.setText("<html><font color=red size=4><b>WARNING!</b></html>");
Image: - We also must you HTML code if we want to add line breaks in the label’s text. For example:
JLabel label = new JLabel("<html><i>This label has <br>two lines</i><html>");
Image:
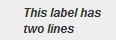
4. Labeling a component
A JLabelis usually used for labeling a component such as a JTextField. If we want to allow the users accessing a text field using shortcut key (mnemonic), use the following code:JTextField textEmail = new JTextField(20); JLabel label = new JLabel("Enter e-mail address:"); label.setLabelFor(textEmail); label.setDisplayedMnemonic('E');Image:

5. JLabel demo program
For reference, we created a Swing program that demonstrates various techniques mentioned when working with JLabelcomponent. The program looks like this: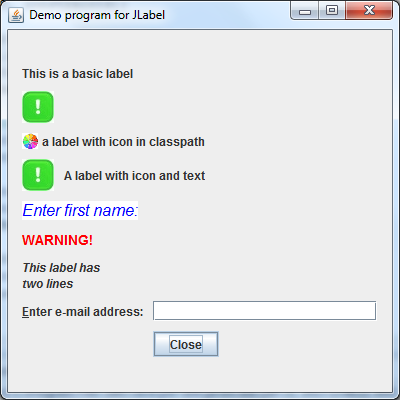
Other Java Swing Tutorials:
- Java Swing Hello World Tutorial for Beginners Using Text Editor
- JFrame basic tutorial and examples
- JPanel basic tutorial and examples
- JTextField basic tutorial and examples
- JButton basic tutorial and examples
- JComboBox basic tutorial and examples
- JCheckBox basic tutorial and examples
- JList basic tutorial and examples
- JTree basic tutorial and examples
About the Author:

Comments
I'm very fine. So I recommend you to follow my tutorials for beginners here: www.codejava.net/java-core-tutorials