Spring Data JPA - How to Sort by Nested Property Examples
- Details
- Written by Nam Ha Minh
- Last Updated on 27 March 2023   |   Print Email
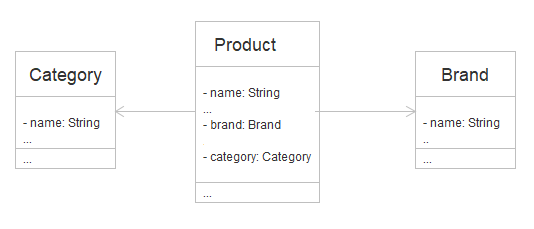
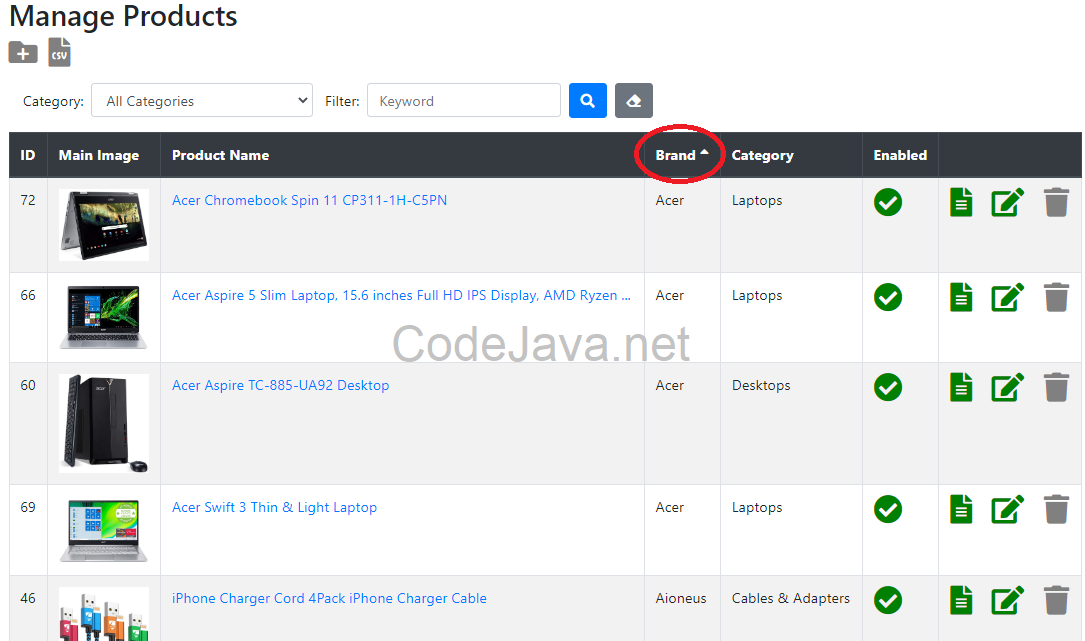
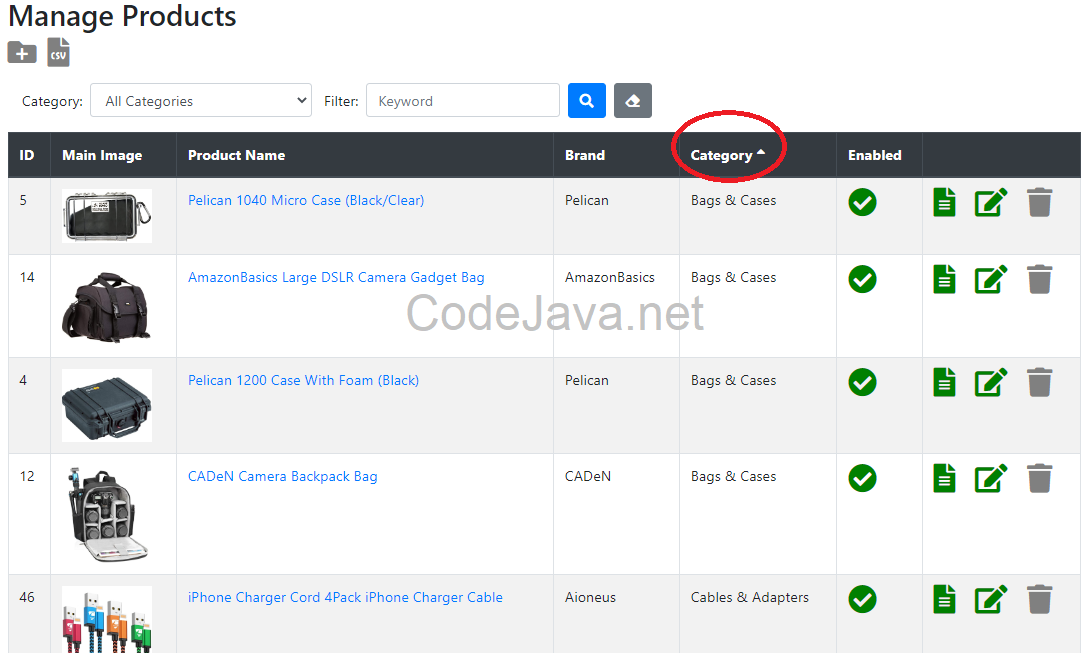
package net.codejava; @Entity @Table(name = "products") public class Product { @Column(length = 256, unique = true, nullable = false) private String name; @ManyToOne @JoinColumn(name = "brand_id") private Brand brand; @ManyToOne @JoinColumn(name = "category_id") private Category category; @Transient public String getShortName() { if (name.length() > 40) { return name.substring(0, 40).concat("..."); } return name; } // other fields, getters and setters... }Code of the Brand class:
package net.codejava; @Entity @Table(name = "brands") public class Brand { @Column(length = 45, nullable = false, unique = true) private String name; // other fields, getters and setters... }Code of the Category class:
package net.codejava; @Entity @Table(name = "categories") public class Category { @Column(length = 128, nullable = false, unique = true) private String name; // other fields, getters and setters... }
package net.codejava; public interface ProductRepository extends PagingAndSortingRepository<Product, Integer> { }And to tell Spring Data JPA to sort a collection of Product entities by brand name, you need to create a Sort object like this:
Sort sort = Sort.by("brand_name").ascending();Spring Data JPA will understand the field name “brand_name” as nested property, i.e. the field name of the Brand object referred to by a Product object. For your reference, below is code example of a Spring Data JPA unit test class that tests sorting products by brand name in ascending order:
package net.codejava; @DataJpaTest @AutoConfigureTestDatabase(replace = Replace.NONE) @Rollback(false) public class ProductRepositoryTests { @Autowired private ProductRepository repo; @Test public void testSortProductsByBrandName() { Sort sort = Sort.by("brand_name").ascending(); Pageable pageable = PageRequest.of(0, 30, sort); Iterable<Product> products = repo.findAll(pageable); products.forEach(p -> { System.out.printf("%-50s - %s\n", p.getShortName(), p.getBrand().getName()); }); } }This would produce the following output (sample data):
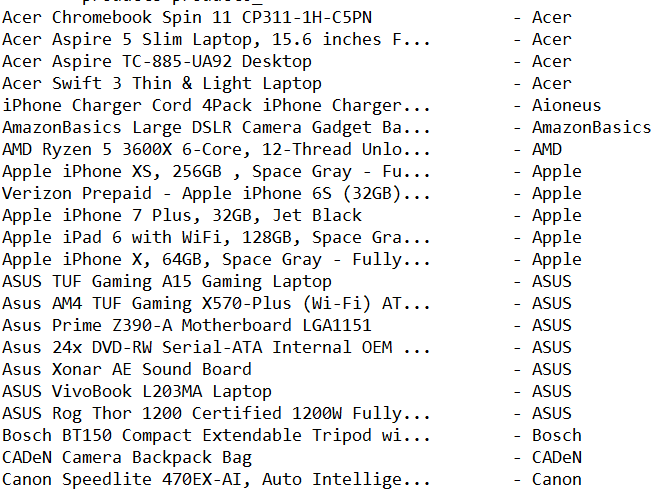
@Test public void testSortProductsByCategoryName() { Sort sort = Sort.by("category_name").descending(); Pageable pageable = PageRequest.of(0, 30, sort); Iterable<Product> products = repo.findAll(pageable); products.forEach(p -> { System.out.printf("%-50s - %s\n", p.getShortName(), p.getCategory().getName()); }); }And sample output:
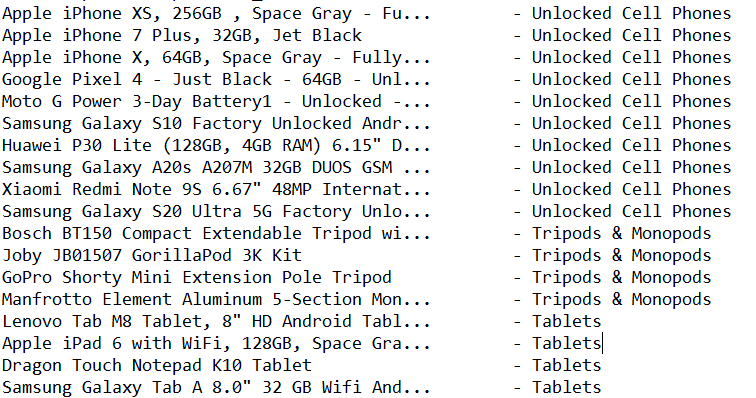
http://localhost/.../products/page/1?sortField=category_name&sortDir=asc
http://localhost/.../products/page/1?sortField=brand_name&sortDir=desc
That’s my tip about sorting by nested property with Spring Data JPA. I hope you found the code examples helpful.Watch the following video to see the coding in action:Related Spring Data JPA Tutorials:
- Spring Data JPA Paging and Sorting Examples
- Spring Data JPA Filter Search Examples
- Spring Boot Full Text Search with MySQL Database Tutorial
- Spring Data JPA EntityManager Examples (CRUD Operations)
- JPA EntityManager: Understand Differences between Persist and Merge
- Understand Spring Data JPA with Simple Example
- Spring Data JPA Custom Repository Example
About the Author:

Comments